Software quality is an essential feature of sustainable software. Clients require software that not only meets their requirements but is also reliable, robust, and performs as expected. When software possesses the necessary quality, the lives of both clients and software developers are not disrupted due to breakdowns.
In software development, to ensure high quality, a multitude of test types are used, ranging from regression, E2E, performance, unit testing, etc. In this article, we are focusing on unit testing. Unlike other types of testing, software engineers are solely responsible for unit testing.
1. What is a unit test?
Unit tests test each component or function of software, especially functions or methods used.
2. Why is unit testing important?
● For early detection of bugs and errors in individual components of the code.
● To ensure that each unit of code functions as planned in isolation, providing a foundation of reliability.
● To act as a safety net during code changes or updates. Developers can confidently refactor or modify code, ensuring that the modifications do not introduce new defects.
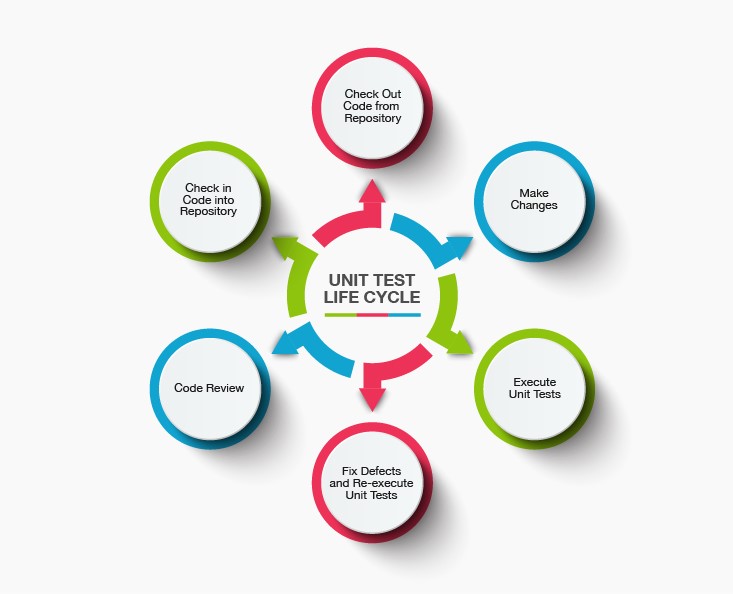
3. How do you plan for unit testing?
- Outline the scenarios and inputs for each unit to be tested clearly. Develop test cases that cover a range of expected and edge cases, ensuring comprehensive coverage of the expected functionality. An important part of designing unit tests is choosing a suitable mocking technology for data mocking.
- Choose appropriate unit testing frameworks and tools that align with the programming languages and development environments.
e.g.
- Java – JUnit
- Python – pytest
- .NET – nUnit
- Javascript – Jasmine
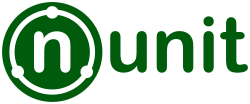
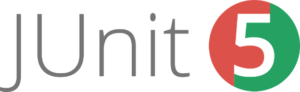
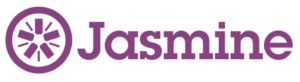
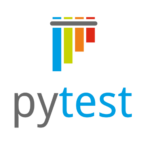
- Set up a dedicated and controlled environment for unit tests. Often, this includes planning for integrating unit tests in the CI/CD pipeline.
4. Essential features of unit testing
- To ensure accuracy, isolating to the smallest testable part is crucial.
- Mocking technologies are employed to simulate external dependencies or complex behavior, allowing tests to focus solely on the component being tested without interference from other parts of the system.
- Code coverage, a vital aspect of unit testing, provides a statistical measure of how much of the codebase is exercised by unit tests. It reveals areas that may need additional test coverage to enhance the overall reliability of the software.
5. ‘Nos’ when planning / designing unit testing
- Avoid writing unit tests that are overly complex or difficult to maintain. Tests should be clear, concise, and focused on specific functionalities to ensure ease of understanding and future modifications.
- Unit tests should be independent and not rely heavily on external systems or data sources. Minimize dependencies to enhance test stability and prevent failures caused by changes in external components.
6. Example of a good unit test
An example of a simple function to perform the addition of two numbers is shared below.
const addNumbers = (a, b) => {
return a + b;
}
Now, let’s write a unit test for this function:
test('adds 1 + 2 to equal 3', () => {
expect(addNumbers(1, 2)).toBe(3);
});
test('adds -1 + 5 to equal 4', () => {
expect(addNumbers(-1, 5)).toBe(4);
});
test('adds 0 + 0 to equal 0', () => {
expect(addNumbers(0, 0)).toBe(0);
});
7. Is 100% unit testing necessary?
Attaining 100% unit testing is impractical due to dependencies on external systems and the complexity of code interactions. Unit tests focus on isolating individual code units, but replicating real-world dependencies can be challenging. Attempting exhaustive coverage becomes impractical as code complexity increases. Common practice recognizes the difficulty and sets a pragmatic goal of maintaining unit test coverage over 90%.
8. Does unit testing make quality testing redundant?
Unit testing does not make other testing strategies redundant; rather, it serves as a fundamental and essential component of a comprehensive testing strategy. Unit testing and other testing strategies (integration testing, system testing, load testing, etc.) have distinct roles, and each contributes to ensuring the overall quality and reliability of software.
Leave a Reply